iOS Firebase Part 1 (Cloud Firestore)
It is a flexible, scalable NoSQL cloud database to store and sync data for client- and server-side development
Getting Started
Step 1 : Create Firebase Project
- Open the Firebase console.
- Click “CREATE NEW PROJECT”. …
- The “Create a project” window opens. …
- The start screen of the Firebase console opens. …
- The “Enter app details” screen opens. …
- Continue the configuration by following the on-screen instruction. …
- Check the server key after the project is created

Project → Contains one or more apps, All apps in the same project use the same Firebase store and cloud storage from firebase backend.
App → An app is a specific application on a single platform — for example an iOS client or a web client. A project can contain several apps, but an app can only be in one project. The slightly confusing thing is that sometimes one codebase is several apps — for example you might have two different variants of an Android app, one free and one paid — each can be a different Firebase app. Or you might have a development App that is separate from the release App.
Step 2: Connect Client app and Install FireStore
- Create app
- Download
GoogleService-Info
- Drag into root folder
- Add this to your
pod ‘Firebase/Firestore’, ‘6.6.0’
podfile pod install
from terminal (see my cocoapods blogs )FirebaseApp.configure()
ondidFinishLaunchingWithOptions
as shown in Figure 1- Run the application


Step 3: Create a Cloud Firestore database
Navigate to the Database section of the Firebase console. You’ll be prompted to select an existing Firebase project. Follow the database creation workflow.
Summary of Cloud FireStore
Cloud FireStore is a document database that means it stores your data in a big tree like structure like normal database but everything is placed into documents and collections.
Now you can think of document something like a dictionary. It’s got key value pairs, which the firebase folks refers to as fields and the value of these fields can be any of things like string , number , binary values , nested json called maps.
Collections are something collections of documents, there are few rules to these things
Rule # 1 → Collections only contains document, no collection of strings
Rule # 2 → Documents can’t contain other documents but they can point to other subcollections
Rule # 3 → The root of your database can only consists of collections
Add Data
As shown in Figure 2 we created a users
collection and created a document inside this collection each will represent different user. red — refers to a document location in a Firestore database and can be used to write, read, or listen to the location. The document at the referenced location may or may not exist. This code will save data to firebase store but what happened we get the authorization error “Missing or insufficient permissions.”
Cloud Firestore contains a set of security rules that determine whether or not a certain action is permitted or not. By default they are setup that nobody can read or write to the database

Solution 1 (Proper Solution) → To add sign In using Firebase auth and then create some proper well thought security rules based on what information I am willing to share with each user
Solution 2 (Not recommended) → Make sample data open to public Go to Firebase console → Go in Database → Rules → Change allow read, write: if
false; to true; → Publish as shown in Figure 3
Note: This completely turns off security for the database! Making it world writable without authentication!!! This is NOT a solution to recommend for a production environment. Only use this for testing purposes.
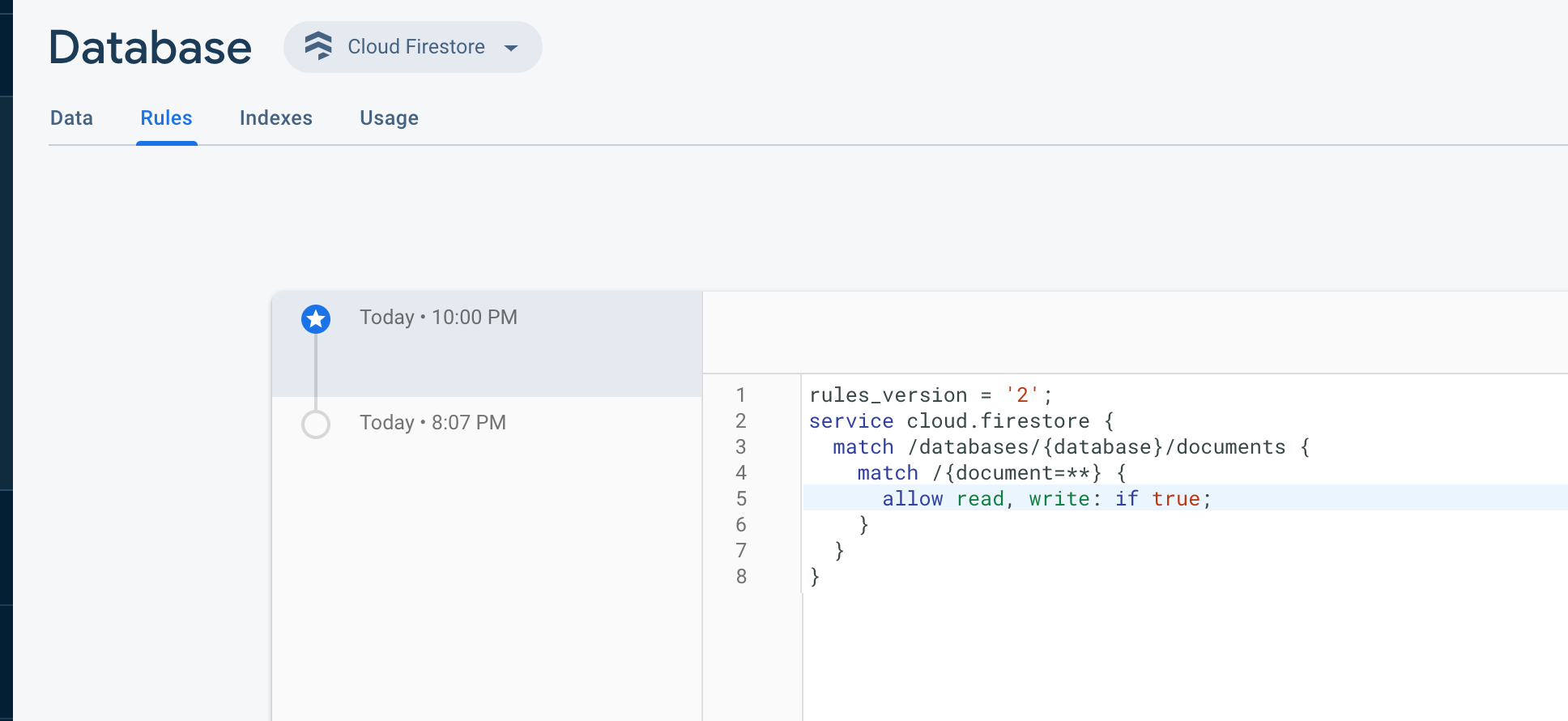
As shown in Figure 4.1 and 4.2 we are successfully stored data into Firestore
Note: addDocument always add a new document to this collection with the specified data, assigning it a document ID automatically.
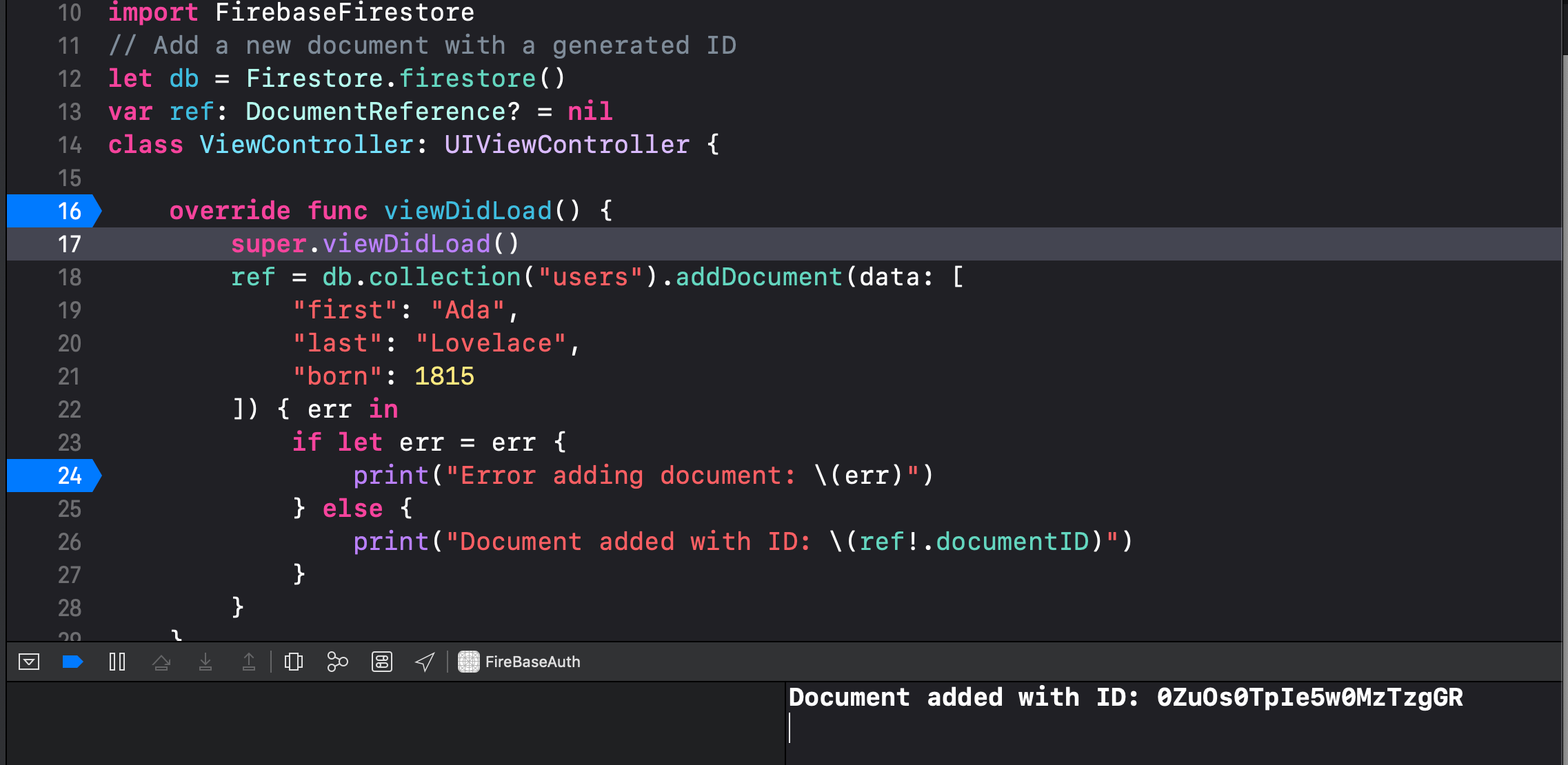

Update Data
As shown in Figure 5 we update data by its document id.
Note: To create or overwrite a single document, use the
set()
method . If the document does not exist, it will be created. If the document does exist, its contents will be overwritten with the newly provided data


As shown in Figure 6 since no user exists in database by using set on document created that user as a document with document id = 123 what we specified and add it to the users collections
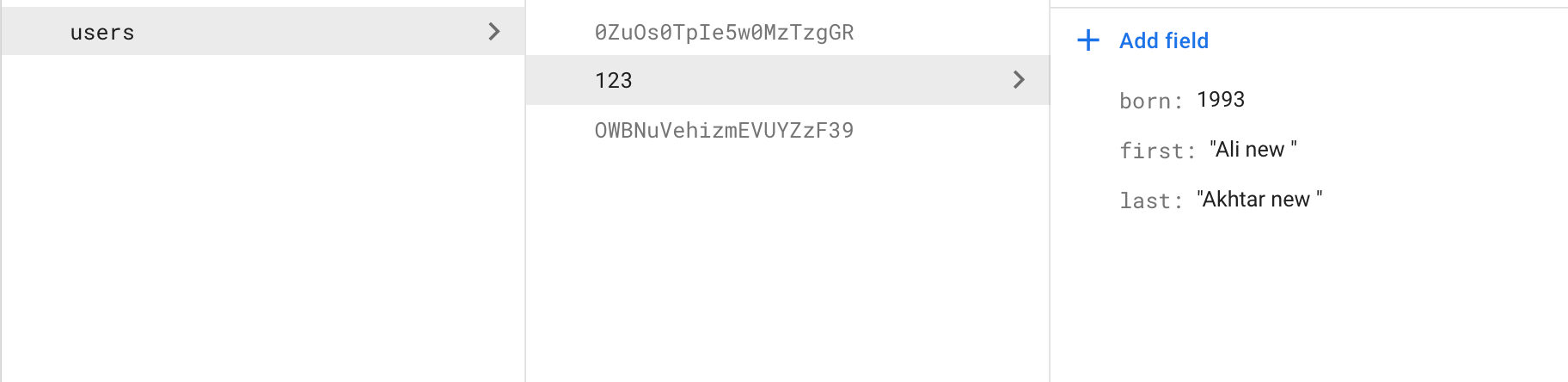
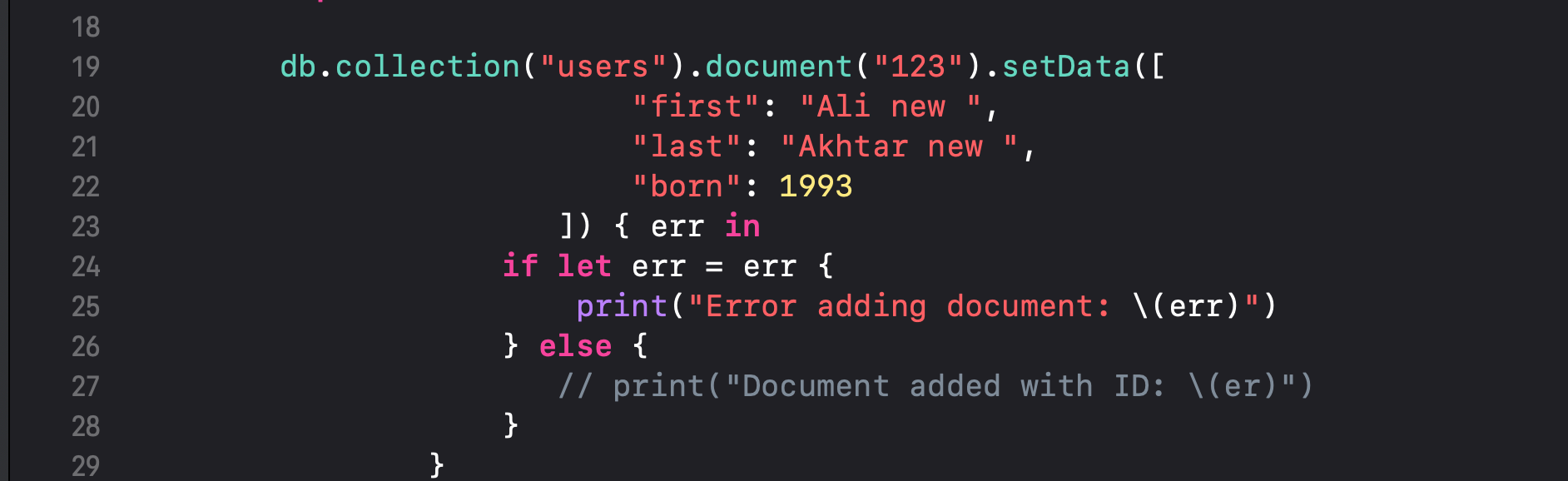
Now if you want to update only the born date
of the user with id = 123
you were thinking to write code like this as shown in Figure 7
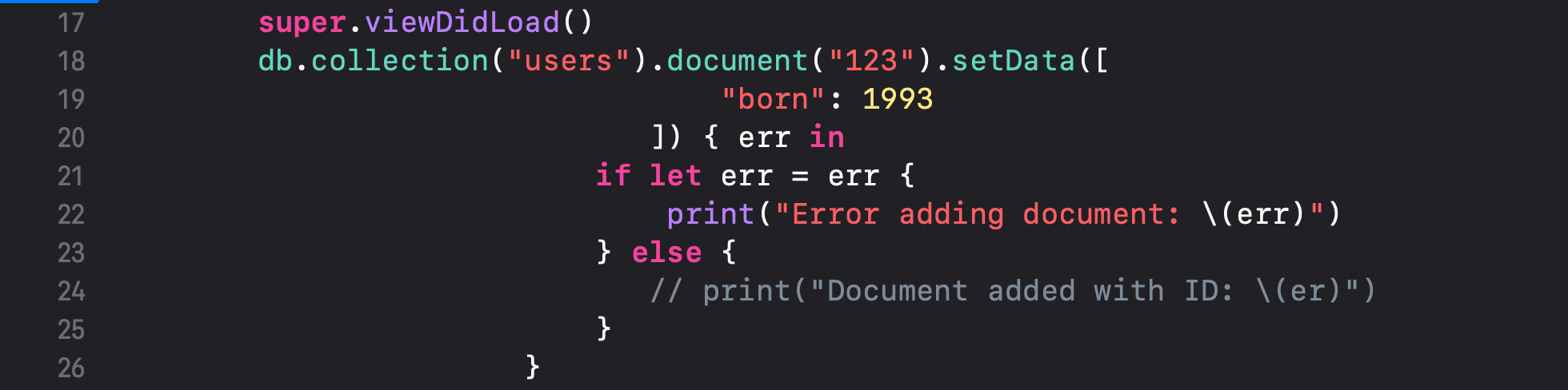
😠we overwrites the document referred to by this `FIRDocumentReference`. The question is how update only specific field. I will show in next command but before that put that as we have before

As shown in Figure 9.1 and 9.2 we update only the fields we specify not the whole document
Note: merge → If the document does not exist, it will be created. If the document does exist, its contents will be merged into the existing document, as follows:
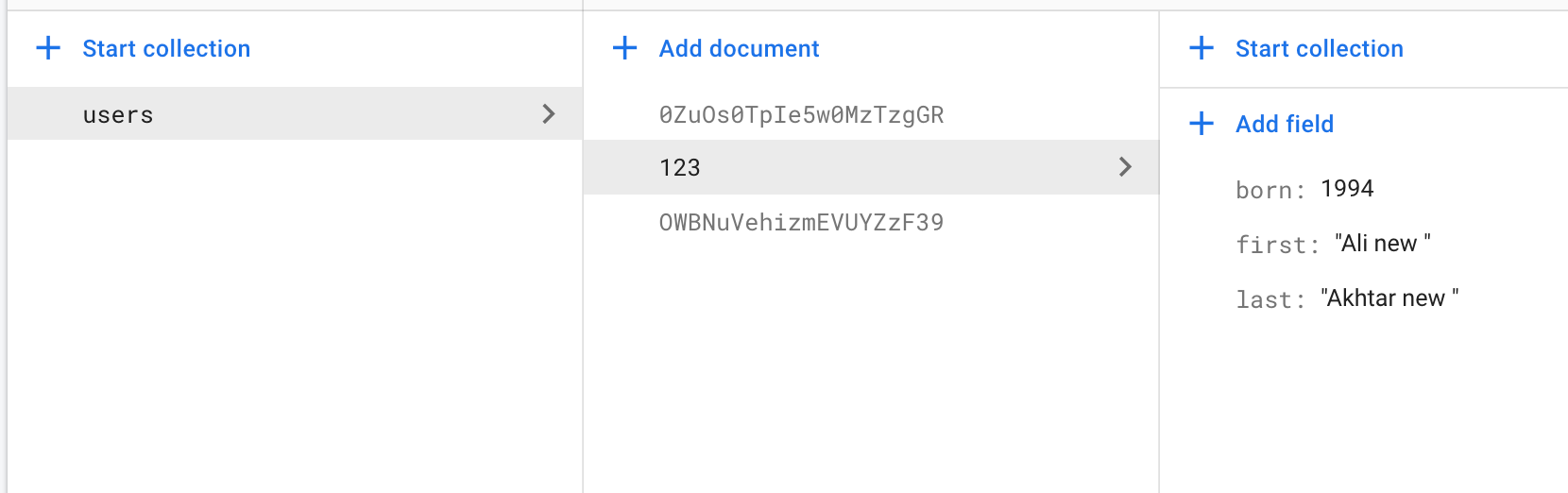

Comments
Post a Comment